Tutorial: Create your own Discord Bot
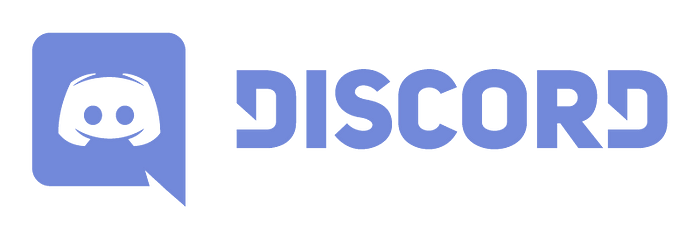
May you have already used an app called Discord. It´s an IRC that is mostly used by gamers and communities. You might already have been able to join guilds with some bots in them. I dont want to get to deep into how bots are used in an IRC. Just think its a kind of automated process.
In this tutorial i will especially explain how you can code your own discord bot with Node JS. If you already have some knowledge in javascript, then this here will be really easy for you, but you dont need to have this knowledge.
Requirements
- You must have Node JS installed on your desktop or hosting.
- You must have a text editor installed. (I recomment Atom)
- You must have a discord account
Lets Get Started
First we will create a folder (it doesnt matter where you will put that) and we will name it “discord-bot” for now.
Now lets create the following files server.js, config.json and package.json
Now let us fill them.
package.json
{
"name": "discord-bot",
"version": "0.0.1",
"description": "My first discord bot.",
"main": "server.js",
"author": "your-name",
"scripts": {
"start": "node server.js"
},
"dependencies": {
"express": "^4.17.1",
"discord.js": "^11.5.1",
"fs": "^0.0.2"
}
}
config.json
{
"bot":"Njk0MDc0MTg4OTEwMTY2MDM2.XoLgtA.c34EBQzKTSKzGJCDNbYnR1JBP4M",
"prefix": "-"
}
To get your token follow this guide on how to setup a Discord app.
server.js
const express = require("express");
const app = express();
const config = require("./config.json");
var server = require("http").createServer(app);app.get("/", (request, response) => {
console.log(Date.now() + " Ping Received");
response.sendStatus(200);
});const listener = server.listen(process.env.PORT, function() {
console.log("Your app is listening on port " + listener.address().port);
});setInterval(() => {
}, 280000);const Discord = require("discord.js");
const {Client, Attachment, Collection, RichEmbed } = require("discord.js");const client = new Client({
disableEveryone: true
});client.on("ready", () => {
console.log(
`Ready to serve on ${client.guilds.size} servers, for ${client.users.size} users.`
);
let activities = [
`${client.guilds.size} Guilds!`,
`${client.channels.size} Channels!`,
`${client.users.size} Members!`
],
i = 0;
setInterval(
() =>
client.user.setActivity(
`btc-help | ${activities[i++ % activities.length]}`,
{ type: "WATCHING" }
),
15000
);
});client.on("message", message => {
if (message.author.bot) return;
// This is where we'll put our code.
if (message.content.indexOf(config.prefix) !== 0) return;const args = message.content.slice(config.prefix.length).trim().split(/ +/g);
const command = args.shift().toLowerCase();if(command === 'hello') {
message.reply("Hey, How are you?");
}
});
client.login(config.bot);
Install Dependencies
Okay, so now our script relies on some node libraries. so we need to them to get installed to.
Open up your command line and navigate inside the project folder, once you’re in, simply install the dependencies by executing the following command:
npm install discord.js express http --save
Run your Bot
Now its time to run our bot! Just type in:
node server.js
Moment of Truth
Now go to your chat server and make sure your bot is connected and online, then type in to the chat box “-hello” it should reply “Hey, How are you?” to you.
If it worked then Conngratulations you have created your first discord bot!
Its just a start but i guess you wouldnt have thought it is so easy.
I hope you learned something and i could help you. Keep going! If you have any questions you can contact me on my social media.